Flutter AnimatedBuilder는 특별히 애니메이션을 쉽게 구성하고 관리할 수 있는 유용한 위젯 중 하나입니다. 이 글에서는 AnimatedBuilder에 대해 알아보고, 어떤 상황에서 사용하면 좋은지, 그리고 실제 예제와 함께 활용 방법을 설명하겠습니다.
Table of Contents
AnimatedBuilder란?
AnimatedBuilder는 Flutter에서 애니메이션을 만들기 위해 사용되는 위젯입니다. 주로 애니메이션 상태를 위젯 트리에 효율적으로 전달하기 위해 사용됩니다. AnimatedBuilder는 Animation 객체와 모든 프레임마다 호출될 콜백 빌더 함수를 사용하여 애니메이션 상태와 그에 따른 UI 변화를 연결합니다.
주요 특징
- 빌더 패턴: Animation의 값이 변경될 때마다 빌더 함수를 호출하여 새로운 UI를 생성합니다.
- 효율성: 필요할 때만 UI를 빌드하므로 성능이 장점입니다.
- 재사용성: 여러 애니메이션 상태를 개별적으로 관리할 수 있다.
Flutter AnimatedBuilder 사용법
다음은 기본적인 AnimatedBuilder를 구현하는 방법입니다.
- animation: 애니메이션 상태를 전달하는 Animation 객체입니다.
- builder: 애니메이션 상태가 변경될 때마다 호출되는 빌더 함수입니다. 빌더 함수는 context와 child를 인자로 받습니다.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatefulWidget { @override _MyAppState createState() => _MyAppState(); } class _MyAppState extends State<MyApp> with SingleTickerProviderStateMixin { late AnimationController _controller; late Animation<double> _animation; @override void initState() { super.initState(); _controller = AnimationController( duration: const Duration(seconds: 2), vsync: this, ); _animation = Tween<double>(begin: 0, end: 300).animate(_controller) ..addListener(() { setState(() {}); }); _controller.forward(); } @override void dispose() { _controller.dispose(); super.dispose(); } @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter AnimatedBuilder Example'), ), body: Center( child: AnimatedBuilder( animation: _animation, builder: (context, child) { return Container( width: _animation.value, height: _animation.value, color: Colors.blue, ); }, ), ), ), ); } }
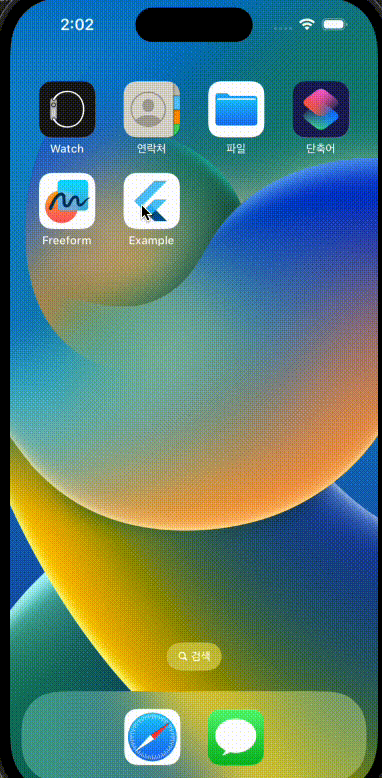
고급 AnimatedBuilder 사용법
Rotation 애니메이션
애니메이션을 통해 회전 효과를 줄 수 있습니다.
import 'dart:math' as math; ... AnimatedBuilder( animation: _controller, builder: (context, child) { return Transform.rotate( angle: _controller.value * 2.0 * math.pi, child: child, ); }, child: Container( width: 100.0, height: 100.0, color: Colors.blue, ), )
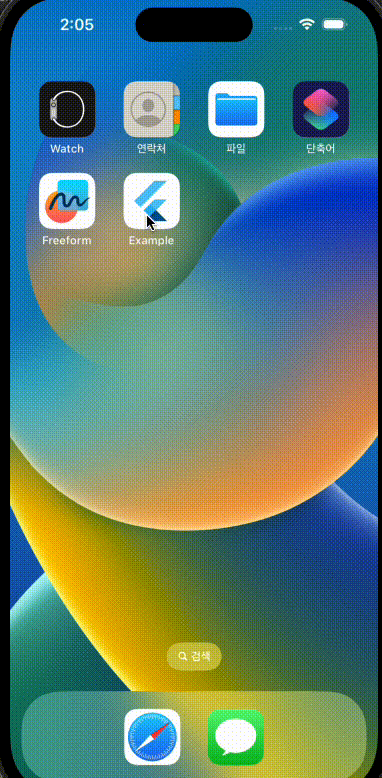
로딩 애니메이션
로딩 화면에서 로딩 중임을 시각적으로 나타내는 애니메이션을 만들 때 유용합니다.
@override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: Center( child: AnimatedBuilder( animation: _controller, builder: (context, child) { return Transform.rotate( angle: _controller.value * 6.28, child: child, ); }, child: Icon(Icons.refresh, size: 50, color: Colors.blue), ), ), ), ); }
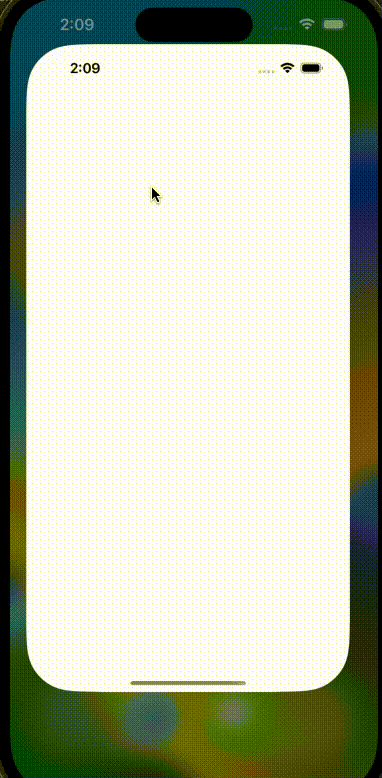
버튼 애니메이션
버튼 클릭 시 버튼의 크기나 색상을 변경하는 애니메이션을 쉽게 구현할 수 있습니다.
@override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( body: Center( child: AnimatedBuilder( animation: _controller, builder: (context, child) { return GestureDetector( onTap: () { _controller.forward(from: 0.0); }, child: Transform.scale( scale: 1.0 + _controller.value, child: child, ), ); }, child: Container( width: 100.0, height: 50.0, color: Colors.orange, child: Center(child: Text('Tap me')), ), ), ), ), ); }
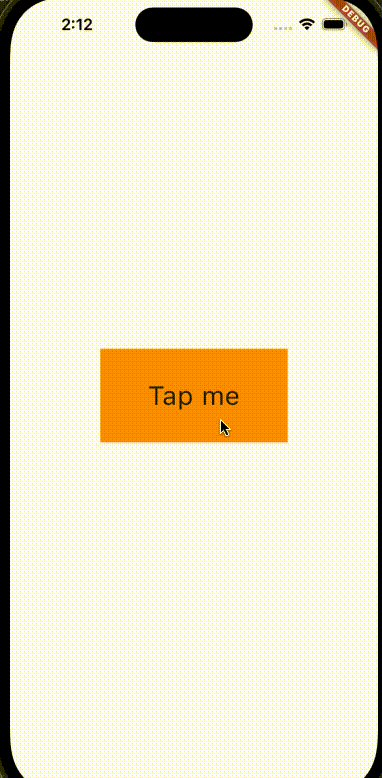