Flutter는 다양한 UI 위젯을 제공하여 개발자들이 복잡한 레이아웃을 쉽게 구현할 수 있게 합니다. 그 중 하나가 Table 위젯입니다. Flutter Table 위젯은 데이터나 콘텐츠를 행과 열로 정렬하여 보여주는 데 유용합니다.
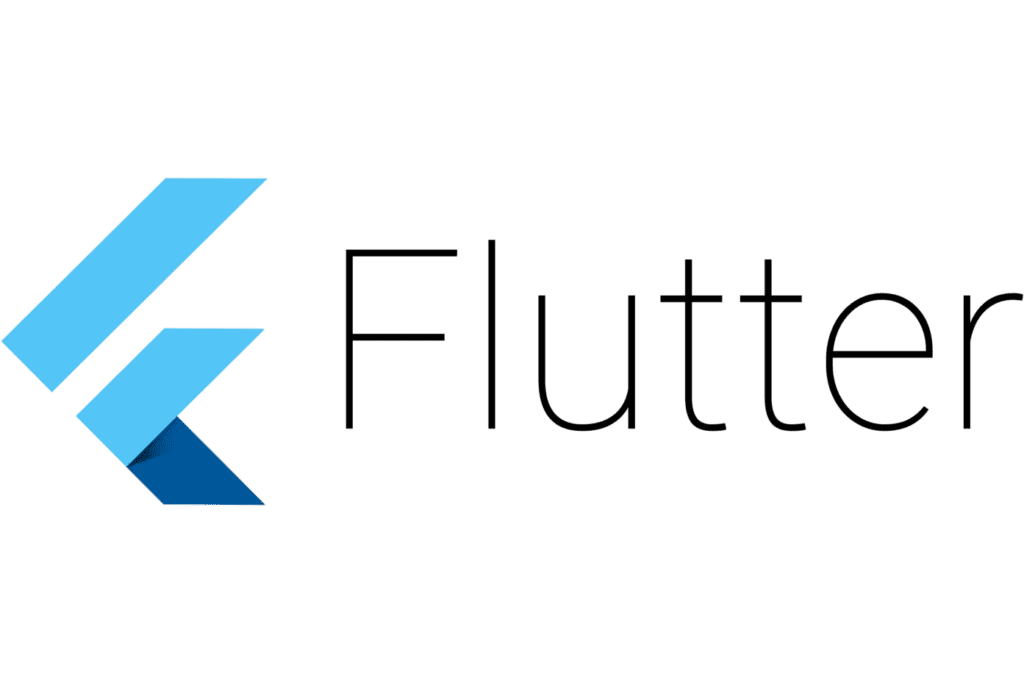
목차
Table 위젯이란?
Table 위젯은 Flutter에서 행과 열을 사용하여 데이터를 정렬하는데 사용하는 레이아웃 위젯입니다. 각 셀은 독립적으로 스타일링 및 정렬이 가능하며, 복잡한 그리드 기반의 UI를 만들 때 매우 유용합니다.
주요 특징
- 행과 열 구성: 테이블을 행과 열을 통해 쉽게 구성할 수 있습니다.
- 셀 스타일링: 각 셀을 독립적으로 스타일링할 수 있어 복잡한 레이아웃을 구현하는 데 유리합니다.
- 고정된 행과 열: 특정 행이나 열을 고정하여 보다 직관적인 데이터 배열을 만들 수 있습니다.
Flutter Table 사용법
다음은 기본적인 Table 위젯을 구현하는 예제입니다.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter Table Example'), ), body: Center( child: Table( border: TableBorder.all(), children: [ TableRow(children: [ TableCell(child: Center(child: Text('Cell 1'))), TableCell(child: Center(child: Text('Cell 2'))), TableCell(child: Center(child: Text('Cell 3'))), ]), TableRow(children: [ TableCell(child: Center(child: Text('Cell 4'))), TableCell(child: Center(child: Text('Cell 5'))), TableCell(child: Center(child: Text('Cell 6'))), ]), ], ), ), ), ); } }
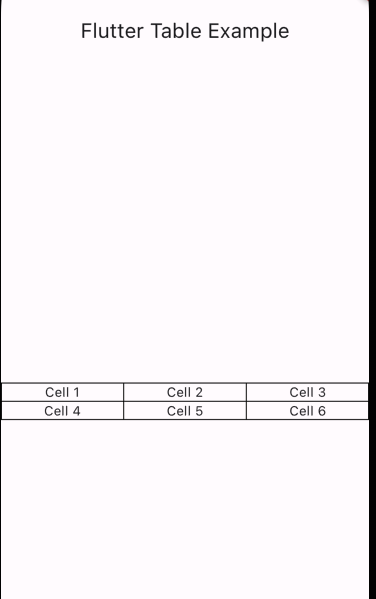
TableRow와 TableCell
TableRow와 TableCell은 Table 위젯의 기본 구성 요소입니다. 각 TableRow는 여러개의 TableCell을 포함할 수 있으며, TableCell은 개별적인 셀을 나타냅니다.
열 너비 및 정렬
Table 위젯에서는 각 열의 너비와 정렬을 지정할 수 있습니다.
Table( border: TableBorder.all(), columnWidths: { 0: FractionColumnWidth(0.2), 1: FractionColumnWidth(0.3), 2: FractionColumnWidth(0.5), }, children: [ TableRow(children: [ TableCell(child: Center(child: Text('Short'))), TableCell(child: Center(child: Text('Medium Text'))), TableCell(child: Center(child: Text('A very long text example to demonstrate column widths'))), ]), TableRow(children: [ TableCell(child: Center(child: Text('Row 2, Col 1'))), TableCell(child: Center(child: Text('Row 2, Col 2'))), TableCell(child: Center(child: Text('Row 2, Col 3'))), ]), ], )
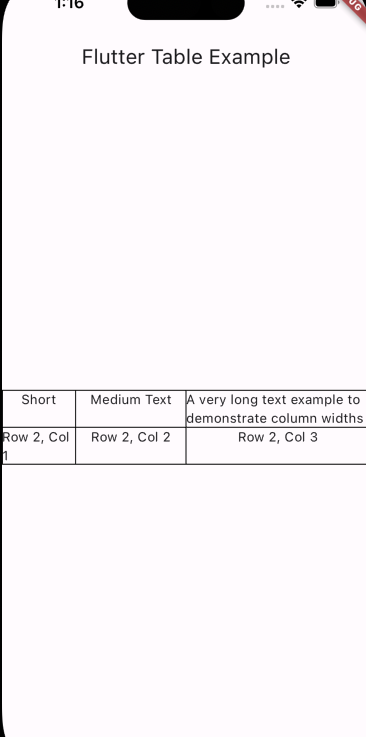
Table 위젯으로 스타일링하기
테이블 셀에 스타일을 적용하여 레이아웃을 좀 더 눈에 띄게 만들 수 있습니다.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter Table Example'), ), body: Center( child: Table( border: TableBorder.all(), children: [ TableRow( decoration: BoxDecoration(color: Colors.grey[200]), children: [ TableCell( child: Padding( padding: EdgeInsets.all(8.0), child: Center(child: Text('Header 1')), )), TableCell( child: Padding( padding: EdgeInsets.all(8.0), child: Center(child: Text('Header 2')), )), TableCell( child: Padding( padding: EdgeInsets.all(8.0), child: Center(child: Text('Header 3')), )), ]), TableRow(children: [ TableCell( child: Padding( padding: EdgeInsets.all(8.0), child: Center(child: Text('Row 1, Col 1')), )), TableCell( child: Padding( padding: EdgeInsets.all(8.0), child: Center(child: Text('Row 1, Col 2')), )), TableCell( child: Padding( padding: EdgeInsets.all(8.0), child: Center(child: Text('Row 1, Col 3')), )), ]), ], )), ), ); } }
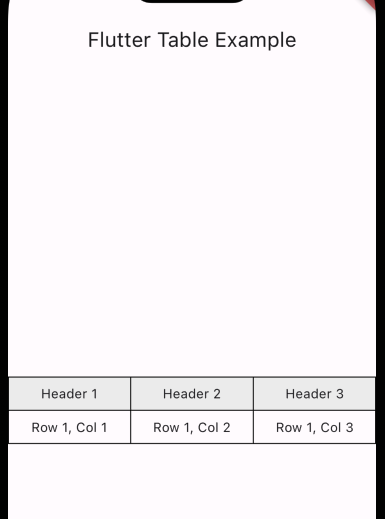