Flutter PageView는 스와이프 제스처를 통해 페이지 간 전환을 간편하게 구현할 수 있도록 하며, 유저 인터페이스를 보다 직관적이고 사용하기 쉽게 만들어 줍니다.
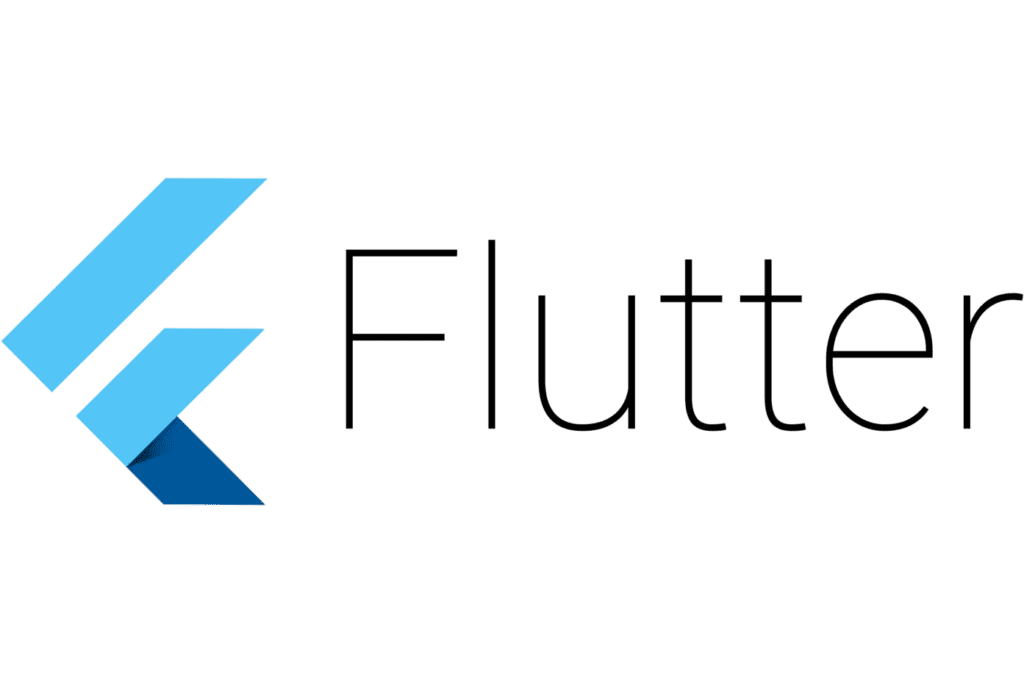
목차
PageView란?
PageView는 플러터의 내장 위젯 중 하나로, 수평 또는 수직으로 여러 페이지를 스와이프할 수 있는 형태로 표시합니다. 이는 프레임워크 내에서 Carousel 또는 주요 콘텐츠를 대체하는 슬라이더로 널리 사용됩니다.
주요 특징
- 스와이프 제스처 지원: 사용자가 손쉽게 페이지 간 전환을 할 수 있도록 합니다.
- 동적 콘텐츠: 리스트와 결합하여 동적으로 생성되는 콘텐츠를 지원합니다.
- 커스텀 페이지 전환 애니메이션: 애니메이션을 커스터마이즈하여 다양한 전환 효과를 제공할 수 있습니다.
Flutter PageView 사용법
다음은 기본적인 PageView를 구현하는 방법입니다.
import 'package:flutter/material.dart'; void main() => runApp(MyApp()); class MyApp extends StatelessWidget { @override Widget build(BuildContext context) { return MaterialApp( home: Scaffold( appBar: AppBar( title: Text('Flutter PageView'), ), body: PageView( children: <Widget>[ Container( color: Colors.red, child: Center(child: Text('Page 1')), ), Container( color: Colors.green, child: Center(child: Text('Page 2')), ), Container( color: Colors.blue, child: Center(child: Text('Page 3')), ), ], ), ), ); } }
PageVide.builder 사용법
페이지 개수가 많거나 동적으로 생성해야 할 경우, PageView.builder
를 사용하는 것이 더 적합합니다.
PageView.builder( itemCount: 10, itemBuilder: (context, index) { return Container( color: index % 2 == 0 ? Colors.blue : Colors.green, child: Center(child: Text('Page $index')), ); }, );
페이지 컨트롤러 사용하기
PageController
를 사용하면 더욱 정교하게 페이지를 제어할 수 있습니다.
import 'package:flutter/material.dart'; void main() => runApp(MyHomePage()); class MyHomePage extends StatefulWidget { @override _MyHomePageState createState() => _MyHomePageState(); } class _MyHomePageState extends State<MyHomePage> { PageController _pageController = PageController(); @override Widget build(BuildContext context) { return Scaffold( appBar: AppBar( title: Text('Flutter PageView with Controller'), ), body: PageView( controller: _pageController, children: <Widget>[ Container(color: Colors.red, child: Center(child: Text('Page 1'))), Container(color: Colors.green, child: Center(child: Text('Page 2'))), Container(color: Colors.blue, child: Center(child: Text('Page 3'))), ], ), floatingActionButton: FloatingActionButton( onPressed: () { _pageController.nextPage( duration: Duration(milliseconds: 300), curve: Curves.easeIn, ); }, child: Icon(Icons.arrow_forward), ), ); } @override void dispose() { _pageController.dispose(); super.dispose(); } }
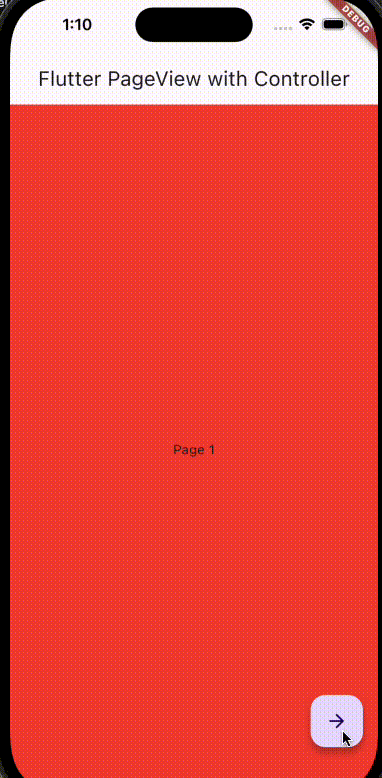